Sample program given below will illustrate the use of CollectionBase
Create a new project in C#, give the name as CollectionBaseDemo and save it to your sample folder. Add a class module to your project and paste the code given below.
using System;
using System.Collections;
using System.Text;
namespace MyCollectionBase
{
public class Product
{
int mintID;
string mstrItemName;
double mdblRate;
public int ID
{
get
{
return (mintID);
}
set
{
mintID = value;
}
}
public string ItemName
{
get
{
return (mstrItemName);
}
set
{
mstrItemName = value;
}
}
public double Rate
{
get
{
return (mdblRate );
}
set
{
mdblRate = value;
}
}
}
public class Products : CollectionBase
{
public Product this[int index]
{
get
{
return (Product )this.List[index];
}
set
{
this.List[index] = value;
}
}
public void Add(Product product)
{
this.List.Add(product);
}
public void Remove(Product product)
{
this.List.Remove(product);
}
}
}
Create a form and name it as frmSample.cs and design it as given in the Fig. given below.
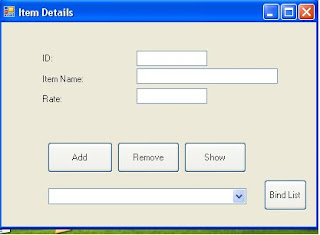
Give the name of buttons as btnAdd, btnRemove and btnShow, button1.
Name of combobox should be cboItems.
Take the code window of form and paste the code below.
using System;
using System.Collections;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
namespace MyCollectionBase
{
public partial class frmSample : Form
{
Products objProducts =null;
public frmSample()
{
InitializeComponent();
objProducts = new Products();
}
private void btnAdd_Click(object sender, EventArgs e)
{
Product objItem = new Product();
objItem.ID = Convert.ToInt32 (textBox1.Text.ToString());
objItem.ItemName = textBox2.Text.ToString();
objItem.Rate = Convert.ToDouble (textBox3.Text.ToString());
objProducts.Add(objItem);
}
private void btnShow_Click(object sender, EventArgs e)
{
int intIndex=0;
try
{
intIndex = Convert.ToInt32(textBox1.Text.ToString());
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
Product objItem = new Product();
objItem = objProducts[intIndex];
if (objItem != null)
{
textBox2.Text = objItem.ItemName;
textBox3.Text = objItem.Rate.ToString();
}
}
private void btnRemove_Click(object sender, EventArgs e)
{
int intIndex=0;
try
{
intIndex = Convert.ToInt32(textBox1.Text.ToString());
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
Product objItem = new Product();
objItem = objProducts[intIndex];
if (objItem != null)
{
objProducts.Remove(objItem);
MessageBox.Show("Item Removed");
}
}
private void button1_Click(object sender, EventArgs e)
{
try
{
cboItems.ValueMember = "ID";
cboItems.DisplayMember = "ItemName";
cboItems.DataSource = objProducts;
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}
}
}